Brick Sizing
How set the brick size.
Base Size
The smallest possible brick is a 1x1 plate. Without side adjustment, each side has the length defined by the parameter baseSideLength, which is 8 mm by default. The height is defined by the parameter baseHeight, which is 3.2 mm by default.
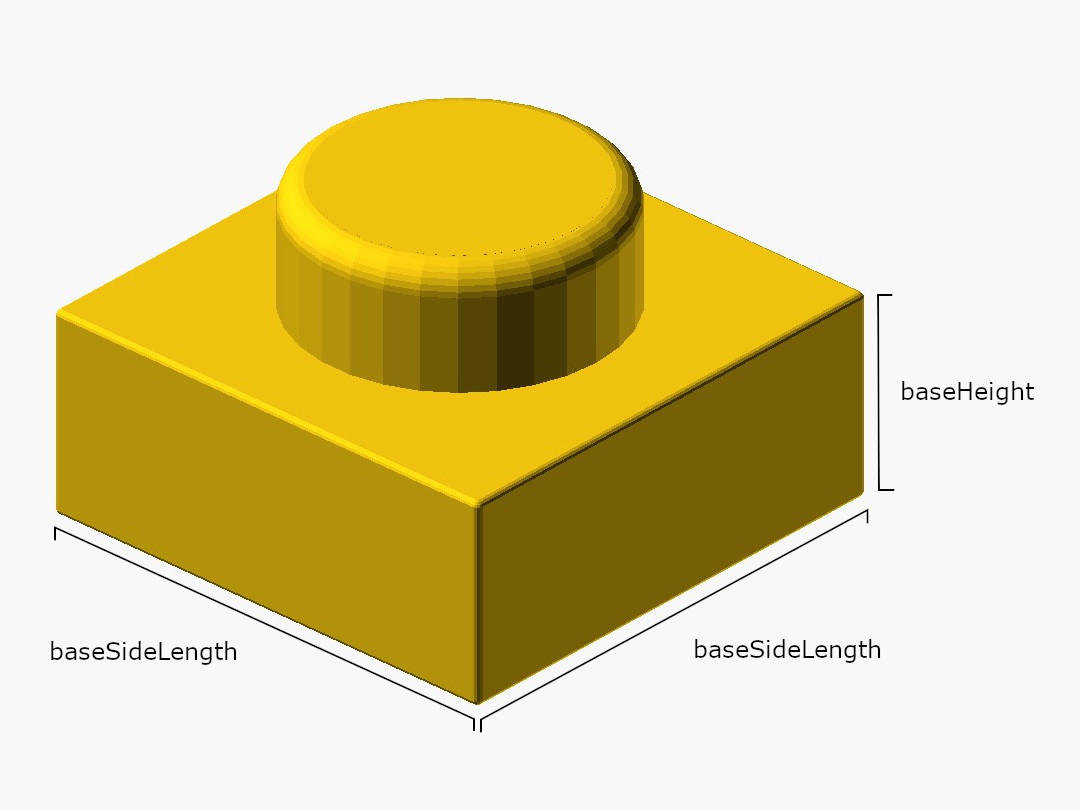
//Import MachineBlocks block() module
use <machineblocks/lib/block.scad>;
//Generate 1x1 Plate without baseSideAdjustment
block(
baseSideAdjustment = 0,
knobSize = 5.0 //Reduce this value if the knobs do not fit into a LEGO brick or only with great difficulty
);
Side Adjustment
Due to inaccuracies during printing and in order to have a certain margin, it is recommended to reduce the length of each side by about 0.1 mm using the baseSideAdjustment parameter. The baseSideAdjustment parameter can be specified as a single value, as a vector of two values or as a vector of four values.
If a single value is specified, the stone is reduced (negative baseSideAdjustment) or enlarged (positive baseSideAdjustment) by this value on each side. If a vector with two values is specified, the first value corresponds to the length adjustment on each side of the X-axis and the second value corresponds to the adjustment on each side of the Y-axis. If four values are specified, the first two values of the vector describe the adjustment at the start or end of the X-axis, the second two values describe the adjustment at the start or end of the Y-axis. By default, the value for baseSideAdjustment is -0.1, i.e. 0.1 mm is subtracted from each side. In this configuration, the side length of a 1x1 panel is therefore effectively 7.8 mm.
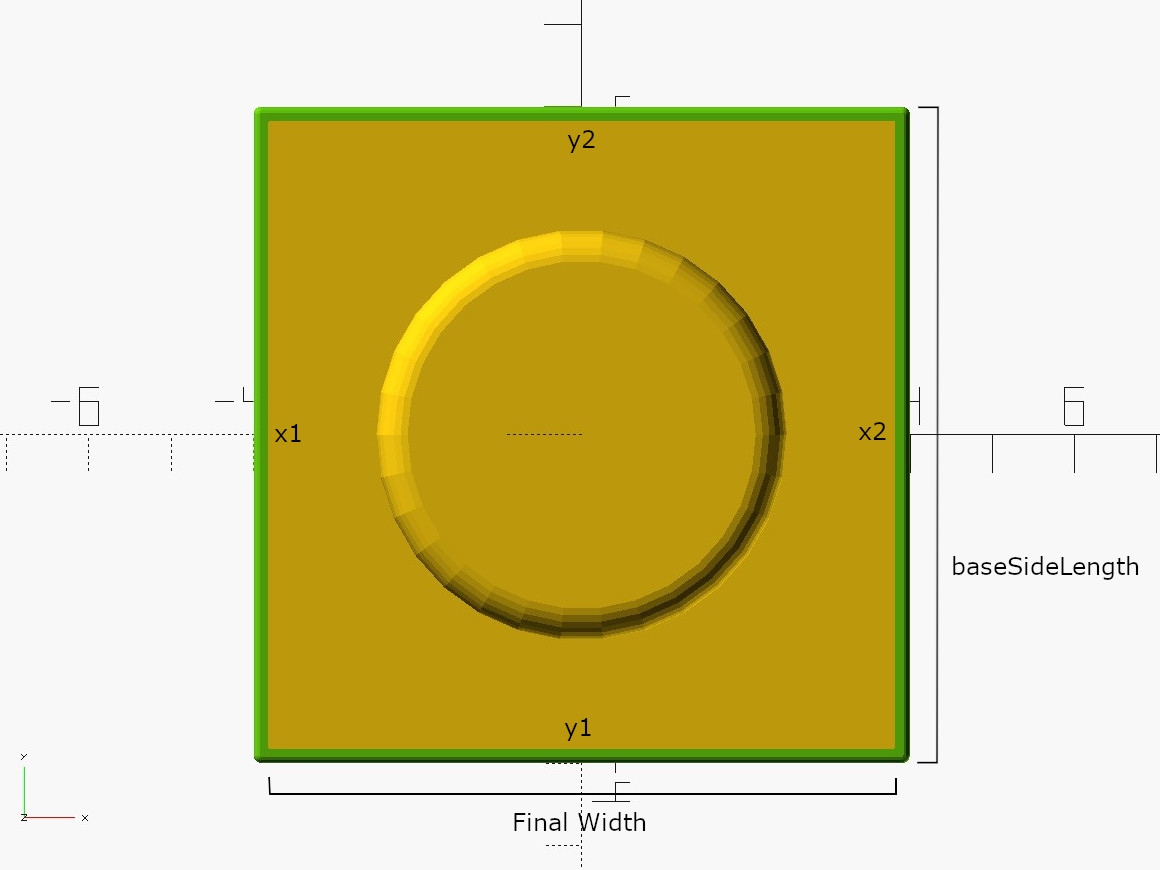
//Import MachineBlocks block() module
use <machineblocks/lib/block.scad>;
//Generate 1x1 Plate with default baseSideAdjustment
block(
baseSideAdjustment = -0.1,
knobSize = 5.0 //Reduce this value if the knobs do not fit into a LEGO brick or only with great difficulty
);
The baseSideAdjustment values are subtracted from (negative baseSideAdjustment) or added to (positive baseSideAdjustment) the respective walls. The size of the recess in the middle always remains the same. If the default value -0.1 is used, the baseSideAdjustment parameter can also be omitted.
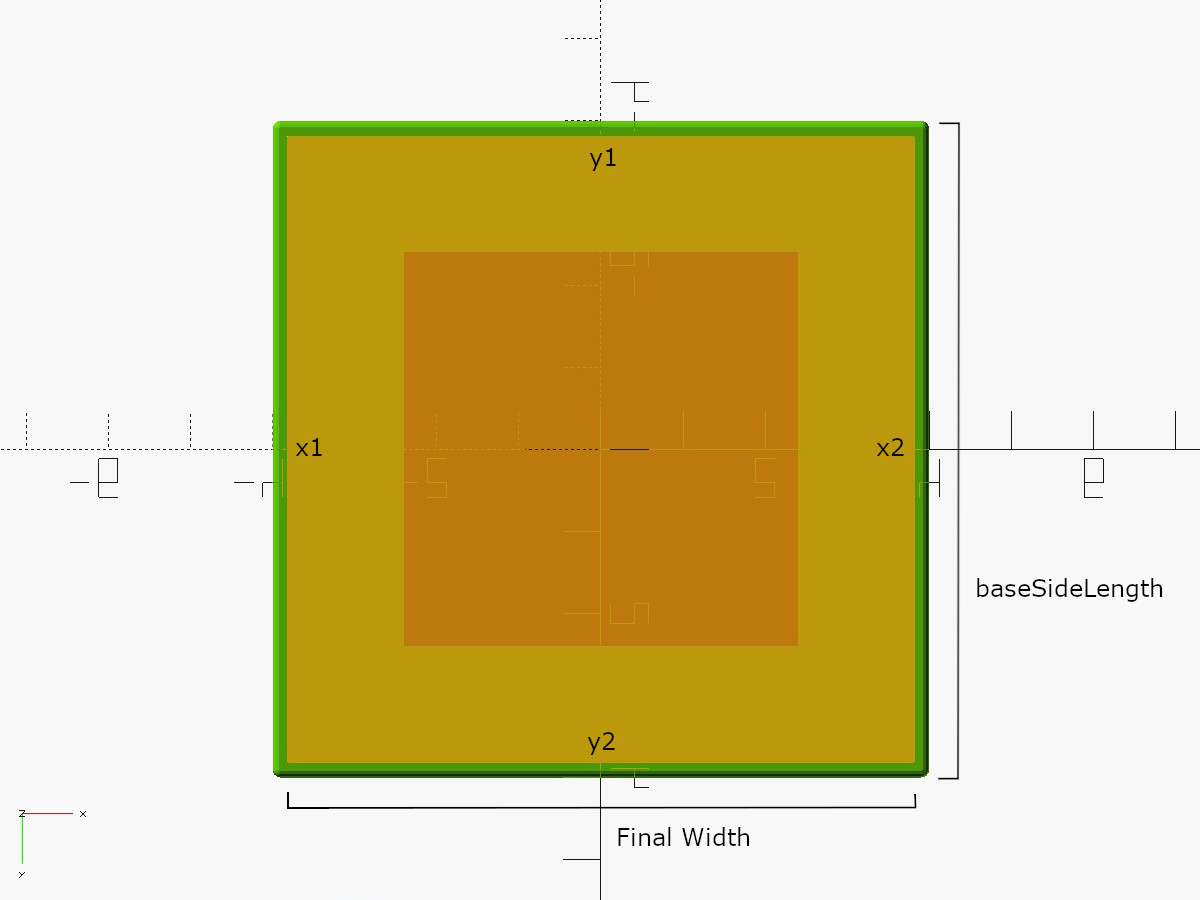
//Import MachineBlocks block() module
use <machineblocks/lib/block.scad>;
//Generate 1x1 Plate with default baseSideAdjustment
block();
Height Adjustment
The height of the brick may have to be reduced due to any inaccuracies in the printing. The baseHeightAdjustment parameter can be used for this. With my Prusa MK3s printer, for example, the height must be reduced by 0.2mm to achieve the height of the original LEGO brick. The effective height of the 1x1 plate is thus reduced to 3.0 mm.
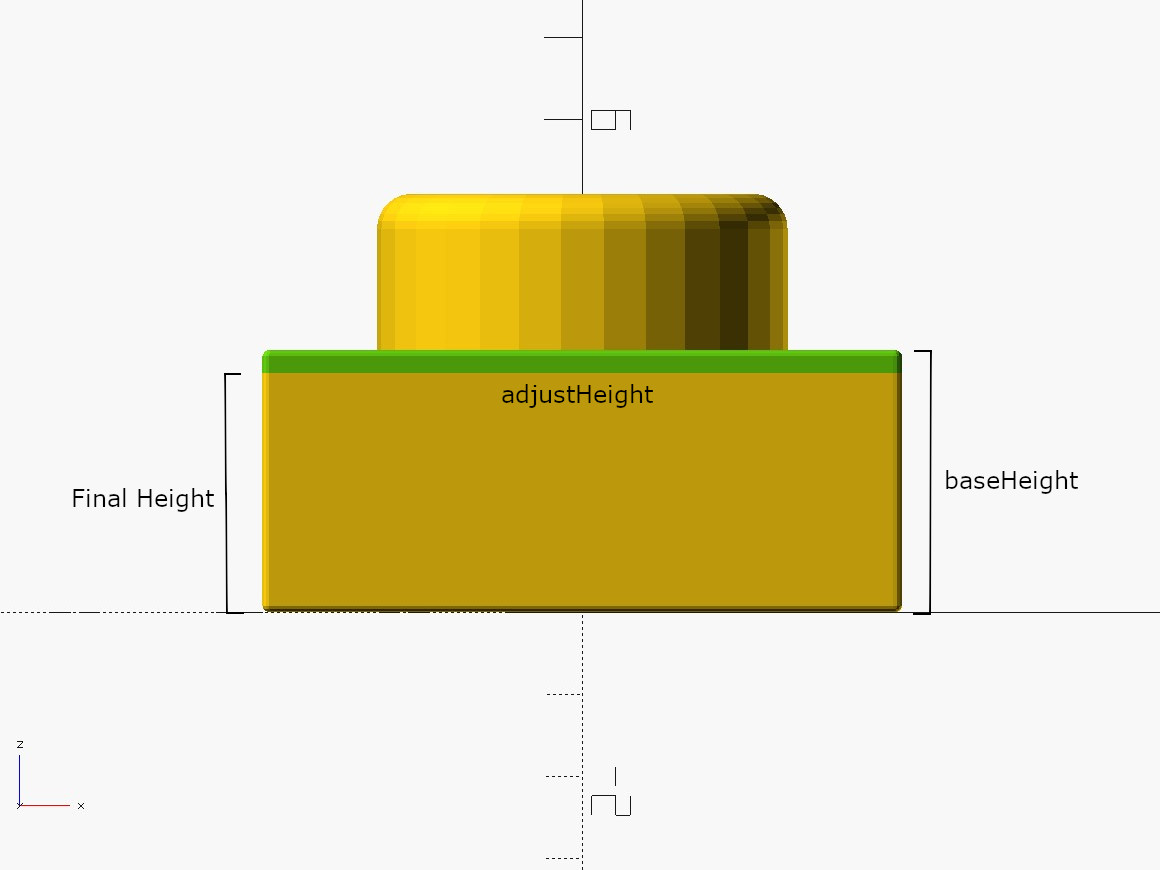
//Import MachineBlocks block() module
use <machineblocks/lib/block.scad>;
//Generate 1x1 Plate with baseHeightAdjustment
block(
baseHeightAdjustment = -0.2,
knobSize = 5.0 //Reduce this value if the knobs do not fit into a LEGO brick or only with great difficulty
);
Base Layers
A standard LEGO brick is 3 times as high as a standard LEGO plate. Therefore, we can use the baseLayers parameter with the value 3 to create a brick. The height adjustment is made at the end, so that the calculated height of 9.6 mm is reduced to 9.4 mm in our example.
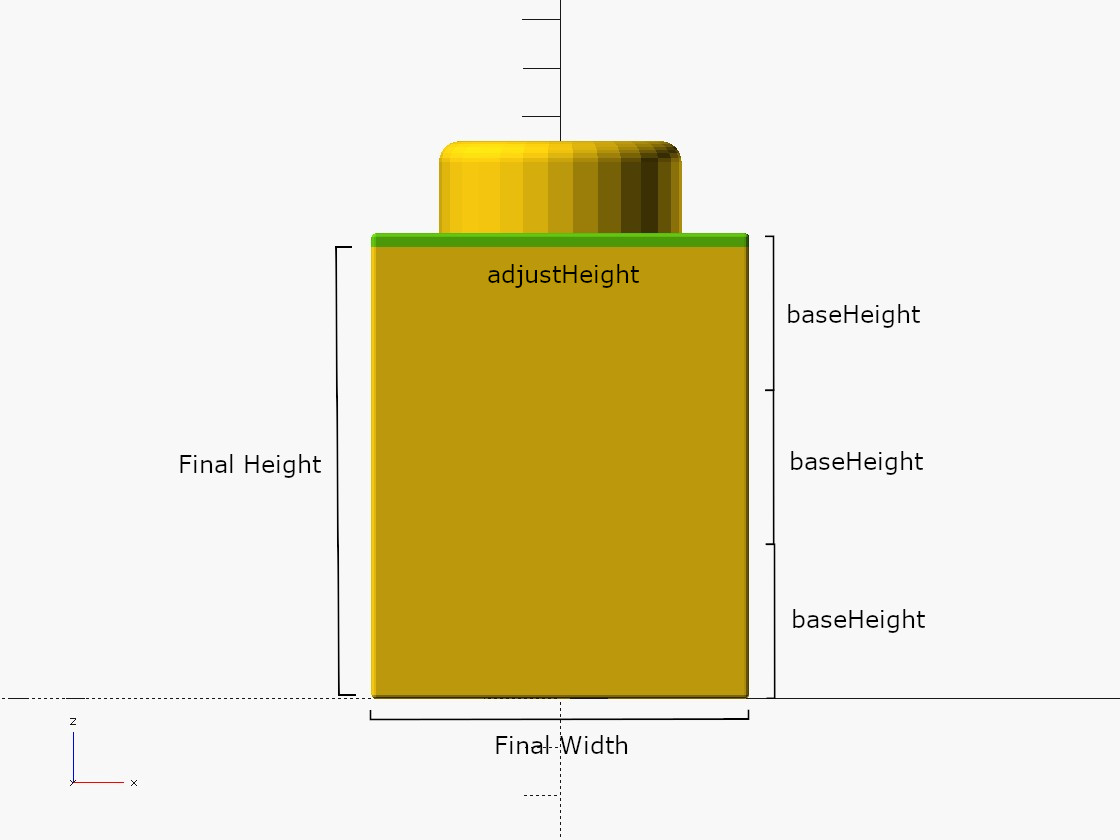
//Import MachineBlocks block() module
use <machineblocks/lib/block.scad>;
//Generate 1x1 Brick with baseHeightAdjustment
block(
baseHeightAdjustment = -0.2,
baseLayers = 3,
knobSize = 5.0 //Reduce this value if the knobs do not fit into a LEGO brick or only with great difficulty
);
Grid
To create horizontally larger bricks, the grid parameter can be used, which is a vector with multiples of the 1x1 brick on the X-axis and Y-axis. A standard 4x2 LEGO brick corresponds to four 1x1 bricks on the X-axis and two 1x1 bricks on the Y-axis. The value for the grid parameter in this example is therefore [4, 2].
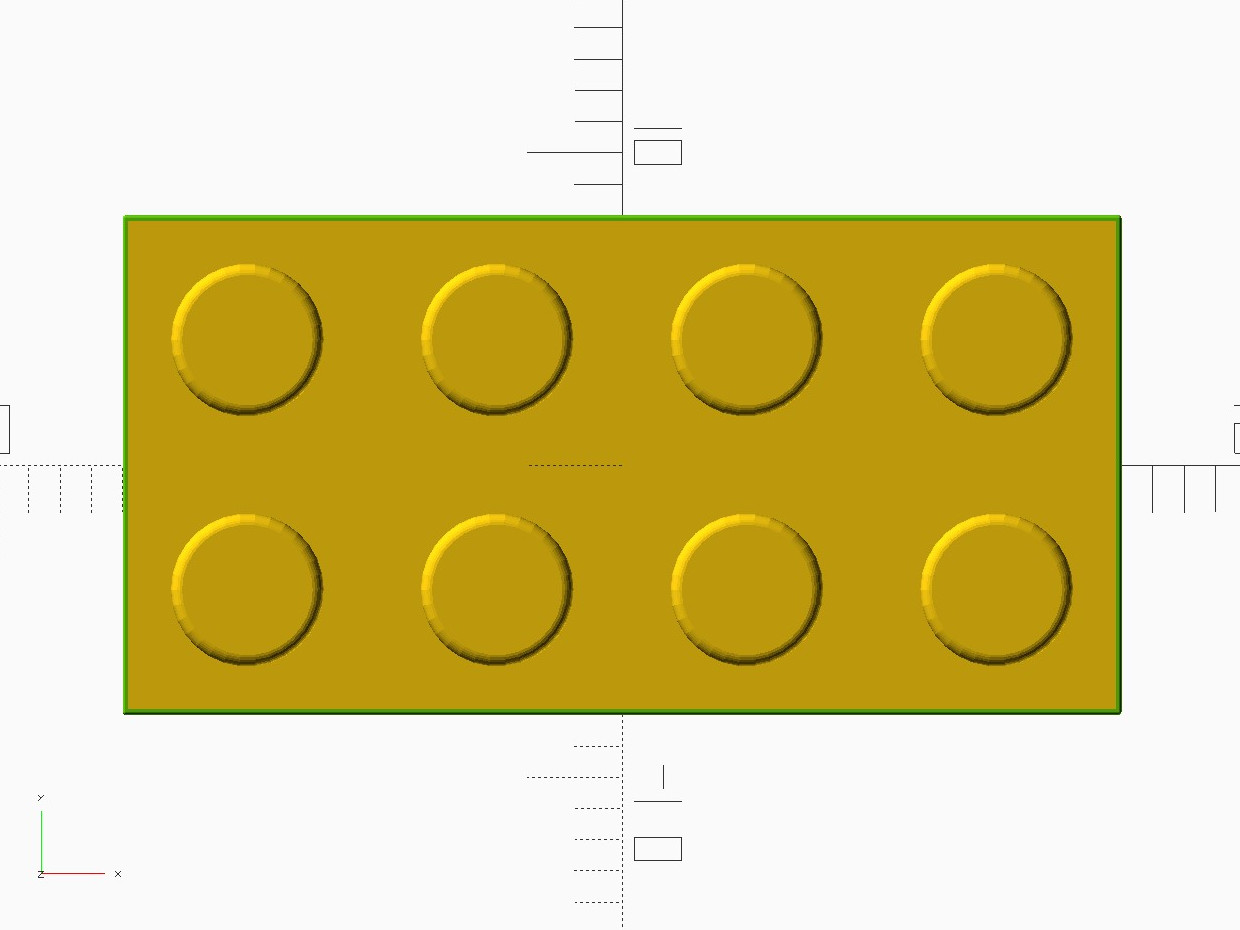
//Import MachineBlocks block() module
use <machineblocks/lib/block.scad>;
//Generate 4x2 Brick with baseHeightAdjustment
block(
baseHeightAdjustment = -0.2,
baseLayers = 3,
grid = [4,2],
knobSize = 5.0 //Reduce this value if the knobs do not fit into a LEGO brick or only with great difficulty
);